Have you ever felt that your C++ code was becoming a tangled mess, riddled with repetition and lacking in flexibility? Are you tired of constantly fighting against the limitations of your own code? The solution to these common coding woes might just lie in the hands of a powerful tool: design patterns. Design patterns are time-tested solutions to recurring problems in software development, offering a blueprint for elegant and efficient code. This guide will empower you to embrace the world of design patterns with a hands-on approach, using practical examples in C++ along with valuable resources to help you navigate your journey.

Image: torob.com
This guide will dive into the fundamentals of design patterns, unlocking their secrets and demonstrating how to master them in the context of C++ programming. We’ll explore the key principles that underpin these patterns, offering a solid foundation for your understanding. You’ll learn how these patterns allow you to enhance your code’s flexibility, maintainability, and scalability, paving the way for cleaner, more robust applications. We’ll investigate patterns like the ubiquitous Singleton, the adaptable Factory, and the versatile Observer, among others. Each pattern will be dissected and explained using practical examples in C++, making their concepts tangible and relatable. By the end of this journey, you’ll be well-equipped to design and implement C++ code that is not only efficient but also elegant, reflecting best practices in the field.
Understanding the Power of Design Patterns
Design patterns are not simply snippets of code to be haphazardly copied and pasted. They represent a crucial design philosophy that emphasizes the importance of reusable solutions to common problems in software development. Imagine a construction site where builders rely on tried-and-true plans to construct sturdy structures. Design patterns are akin to these blueprints, providing a reliable framework for building software that meets specific needs.
The beauty of design patterns lies in their adaptability. They can be tailored to suit various contexts, making them a valuable asset for programmers of all levels. Whether you’re building a simple utility application or a complex enterprise software system, design patterns can provide the structure and flexibility you need to succeed.
Core Principles of Design Patterns
Before delving into the fascinating world of specific patterns, let’s explore the overarching principles that guide their design and application. These principles form the bedrock of effective software design, ensuring that your code is not only functional but also maintainable, reusable, and extensible.
- Abstraction: Design patterns utilize abstraction to hide complex details and provide a simplified interface for interaction. This promotes code modularity and reduces the burden on developers who interact with your code.
- Encapsulation: Data and functionality are carefully bundled together, ensuring that internal details remain hidden, protecting the code from unwanted external intervention. This maintains data integrity and promotes code reusability.
- Polymorphism: This powerful concept allows objects of different types to be treated as objects of a common type. It fosters flexibility and adaptability, making your code more robust and easy to modify.
- Inheritance: This principle promotes code reusability by allowing new classes to inherit traits and behaviors from existing ones. Inheritance helps maintain a consistent code structure and allows for the extension of functionality without reinventing the wheel.
- Composition: This principle emphasizes the building of complex objects from simpler ones. Composition offers a more flexible approach than inheritance, allowing objects to be tailored to specific needs.
Essential Design Patterns in C++
Now, let’s dive into some of the most common and powerful design patterns used in C++ development. Each pattern will be introduced with a brief description, followed by a practical C++ example, highlighting its implementation and benefits.
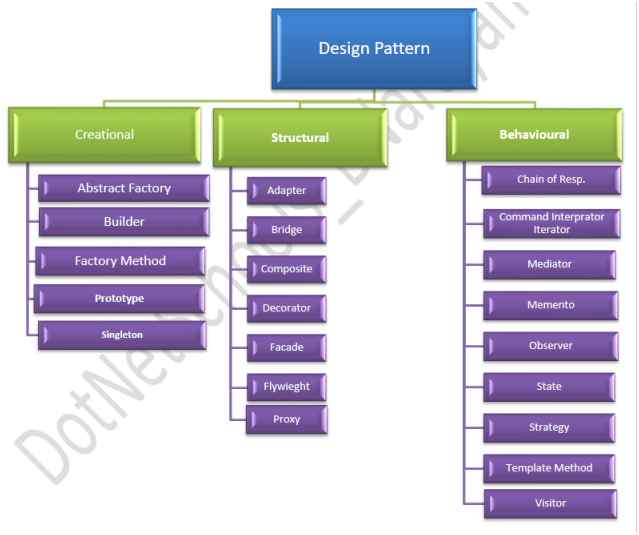
Image: dsigngo.blogspot.com
Singleton
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. This pattern is often used for managing resources, configurations, or logging.
#include <iostream>
class Logger
private:
Logger() // Private constructor to prevent direct instantiation
static Logger* instance;
public:
static Logger* getInstance()
if (instance == nullptr)
instance = new Logger();
return instance;
void logMessage(const std::string& message)
std::cout << "Log Message: " << message << std::endl;
;
Logger* Logger::instance = nullptr;
int main()
Logger* logger1 = Logger::getInstance();
Logger* logger2 = Logger::getInstance();
logger1->logMessage("This is a log message.");
logger2->logMessage("Another log message from the same instance.");
return 0;
In this example, the Logger
class restricts the creation of multiple instances using a private constructor. The getInstance
function acts as the sole entry point, ensuring that only a single instance of Logger
is ever created.
Factory
The Factory pattern provides an interface for creating objects, but it lets subclasses decide which class to instantiate. This pattern promotes loose coupling and flexibility.
#include <iostream>
class Shape
public:
virtual void draw() = 0;
;
class Circle : public Shape
public:
void draw() override std::cout << "Drawing a circle" << std::endl;
;
class Square : public Shape
public:
void draw() override std::cout << "Drawing a square" << std::endl;
;
class ShapeFactory
public:
Shape* createShape(const std::string& type)
if (type == "circle")
return new Circle();
else if (type == "square")
return new Square();
else
return nullptr;
;
int main()
ShapeFactory factory;
Shape* circle = factory.createShape("circle");
Shape* square = factory.createShape("square");
if (circle != nullptr)
circle->draw();
if (square != nullptr)
square->draw();
return 0;
In this example, the ShapeFactory
class provides a consistent interface for creating objects of various shapes. The createShape
function dynamically selects the appropriate shape based on the input string, allowing the code to be easily extended for new shapes without modifying the factory class itself.
Observer
The Observer pattern defines a one-to-many dependency between objects, where a change in one object (the “subject”) triggers a notification to its dependents (the “observers”). This pattern promotes loosely coupled systems, allowing for flexibility in how changes are handled.
#include <iostream>
#include <vector>
class Subject
private:
std::vector<Observer*> observers;
public:
void attach(Observer* observer)
observers.push_back(observer);
void detach(Observer* observer)
observers.erase(std::remove(observers.begin(), observers.end(), observer),
observers.end());
void notifyObservers()
for (auto observer : observers)
observer->update(this);
;
class Observer
public:
virtual void update(Subject* subject) = 0;
;
class ConcreteObserver1 : public Observer
public:
void update(Subject* subject) override
std::cout << "ConcreteObserver1: Reacted to the subject's change."
<< std::endl;
;
class ConcreteObserver2 : public Observer
public:
void update(Subject* subject) override
std::cout << "ConcreteObserver2: Reacted to the subject's change."
<< std::endl;
;
int main()
Subject subject;
ConcreteObserver1 observer1;
ConcreteObserver2 observer2;
subject.attach(&observer1);
subject.attach(&observer2);
subject.notifyObservers();
return 0;
In this example, the Subject
class maintains a list of Observer
objects. When the Subject
undergoes a change, it notifies all its observers using the notifyObservers
function. The observers, like ConcreteObserver1
and ConcreteObserver2
, react to the change in their own ways. This pattern promotes decoupling, as the Subject
doesn’t need to know the specific types of observers or how they respond to changes.
Mastering Design Patterns with Resources
Understanding design patterns is crucial, but becoming a master requires hands-on practice and exploration. Here are some invaluable resources to deepen your knowledge:
-
“Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma, et al: This classic book is considered the definitive guide to design patterns, providing a comprehensive exploration of 23 patterns.
-
“C++ Design Patterns” by James O. Coplien: A dedicated resource focused on design patterns in the context of C++, offering insights and examples specific to this programming language.
-
Online Tutorials and Courses: Platforms like Codecademy, Coursera, and Udemy offer excellent courses on design patterns, covering both theoretical concepts and practical implementation in C++.
-
Open-Source Projects: Dive into open-source C++ projects hosted on platforms like GitHub. Analyze how experienced developers have implemented design patterns within real-world applications, gaining valuable insights from their approach.
Hands-On Design Patterns With C++ Pdf
Unlocking Your Programming Potential with Design Patterns
By understanding design patterns, you’ve taken the first step towards writing cleaner, more maintainable, and scalable C++ code. Invest in learning and practicing these patterns, and you’ll find your software development skills soaring to new heights. Design patterns are not just theoretical concepts but practical tools that can revolutionize your approach to coding. Embrace them, and you’ll unlock your full potential as a C++ programmer.
Don’t just read about design patterns – actively experiment with them! Create your own projects using these principles and watch as your code transforms into a masterpiece of organization and elegance. Share your experiences with others in the programming community, and help spread the power of design patterns to future generations of developers. The journey to mastering these patterns is ongoing, but with dedication and practice, you can unlock a world of possibilities in your C++ development endeavors.