Introduction
Imagine you’re building a house. You have a pile of bricks, each representing a complex object in your C++ program. You need to move these bricks from one location to another, creating your house. Traditional C++ copying would be like physically moving each brick, a painstaking process. Now imagine a magic spell that lets you instantly transport the bricks, leaving empty spaces behind. That’s the essence of move semantics: a powerful technique that allows you to relocate data efficiently, avoiding unnecessary copying.
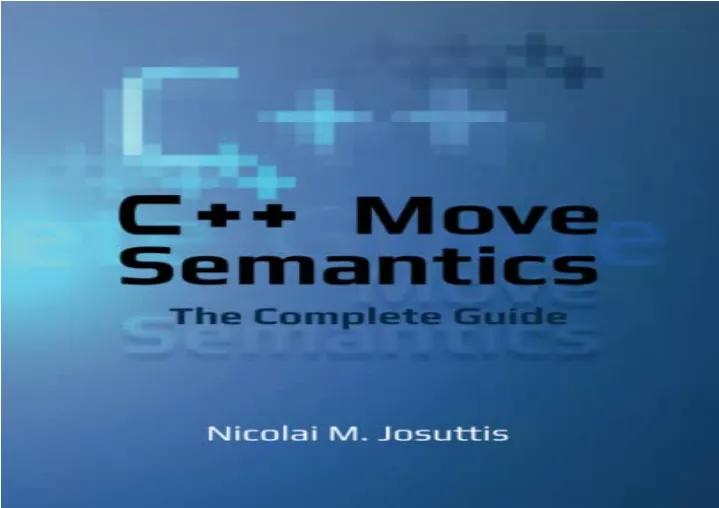
Image: www.slideserve.com
Move semantics, a fundamental concept in modern C++, revolutionizes how we handle object construction and transfer. This guide delves deep into the intricacies of move semantics, empowering you to harness its efficiency and optimize your C++ code for performance.
Understanding Move Semantics
The Need for Efficiency
In the realm of C++, copying objects can be costly, especially for large, complex data structures. This arises from the potential need to allocate new memory and meticulously duplicate every byte of data. Traditional copy constructors, while ensuring data integrity, often expend significant resources in this process.
Move Semantics: The Magic Spell
Move semantics addresses this efficiency bottleneck by enabling a more efficient transfer of resources. Instead of copying data, move semantics employs resource transfer, essentially “stealing” the resources of the source object and reusing them in the destination object. This eliminates the copying overhead, resulting in significant performance gains.

Image: savas.me
Key Concepts:
To fully grasp move semantics, it’s vital to understand its core components:
- Move Constructors: These specialized constructors accept objects by **rvalue reference** (denoted by &&) and transfer resources from the source object to the destination object. After the move, the source object is left in a valid but unspecified state, often ready to be destroyed.
- Move Assignment Operators: Similar to move constructors, these operators take rvalue references as arguments, transferring resources from the source object to the destination object. The source object, after the move, is typically in a valid state, but its contents may be undefined.
- Rvalue References: These references can bind only to temporary objects or the result of a function call. This distinction is crucial, as it enables the compiler to infer when to use move operations over traditional copy operations.
The Power of Move Semantics in Practice
Consider the example of a **std::vector**. When you copy a vector, the entire array of elements is copied. This can be a substantial overhead if the vector holds many elements. With move semantics, however, the resources of the source vector are transferred to the destination vector, effectively “stealing” the data. The original vector is left empty, ready for destruction. This operation is significantly faster than its copy counterpart.
Boosting Performance with Move Semantics
Optimizing Resource Management
Move semantics are particularly advantageous when dealing with resources that are expensive to copy. Imagine a vector of large images—copying such a vector would be computationally demanding. Move semantics allows you to transfer ownership of the image data to a new vector, significantly reducing copying time.
Reducing Memory Footprint
By avoiding unnecessary copies, move semantics contribute to a smaller memory footprint. This is especially beneficial when working with large data structures or when memory resources are limited. For example, moving data between different layers of an application (e.g., from network processing to a database) can minimize memory overhead.
Leveraging the Power of `std::move`
The `std::move` function is your ally in leveraging move semantics. It takes an lvalue (a non-temporary object) and casts it to an rvalue reference. This effectively allows you to use an existing object as a temporary and trigger move operations. Note: This does not physically move the object; it simply tells the compiler to treat it as if it were movable.
Practical Tips and Expert Advice
Embracing Move Semantics in Your Code
To effectively harness move semantics, follow these guidelines:
- Use `std::move` Judiciously: When you want to transfer ownership of an object to another without copying, use `std::move` to cast the object to an rvalue reference.
- Provide Move Constructors and Assignment Operators: Define move constructors and assignment operators for your custom classes to enable efficient resource transfer during object construction or assignment.
- Prefer `std::unique_ptr` and `std::shared_ptr`: These smart pointers are designed with move semantics in mind and offer efficient resource management. Learn about their move-aware nature and leverage their capabilities.
Analyzing and Optimizing Performance
To measure the performance benefits of move semantics, use a profiler. This tool provides valuable insights into your program’s execution, highlighting areas where move semantics can contribute to optimization. By identifying bottlenecks, you can selectively apply move operations to maximize performance.
FAQ
Q: What is the difference between move semantics and copy semantics?
A: While copy semantics replicates data, move semantics transfers ownership of resources, effectively “stealing” them from the source object. This avoids the overhead of copying, leading to increased performance.
Q: When is move semantics necessary?
A: When dealing with large objects or resources that are costly to copy, move semantics become essential for efficient resource management and optimized performance. If memory consumption is a concern, move semantics can help you reduce your program’s footprint.
Q: How do I know if move semantics is being used?
A: You can check the compiler output or use a debugger to inspect the code execution flow. Look for calls to move constructors and assignment operators instead of regular copy operations.
Q: What are the potential drawbacks of move semantics?
A: While move semantics offer significant benefits, there are some potential drawbacks. Firstly, the source object may be in an unspecified state after a move. This requires careful consideration in your code design. Secondly, relying on move operations for every object might not always be the most efficient approach. A performance analysis can guide you in determining the most effective strategy for your application.
C++ Move Semantics – The Complete Guide Pdf
Conclusion: Unleash the Power of C++ Move Semantics
Move semantics is a powerful feature of modern C++ that empowers you to optimize resource management and boost your program’s performance. By grasping the principles of move semantics, you can create more efficient and performant C++ applications.
Are you ready to leverage move semantics to streamline your C++ code? Share your thoughts and experiences in the comments below!